2020. 3. 3. 21:42ㆍ카테고리 없음
This example shows how to format an XML file. When the computer processes an XML file, it doesn’t care about formatting. It doesn’t need indentation and new lines to make the file look nice.
As long as the file is properly constructed, the file can be run all together in one long line as in:AlbertAnders11111BettyBeach22222ChuckCinder33333Formatting an XML document for human consumption isn’t hard, but it is confusing. The following code assumes you have created an XML document object as described in the example. The following code formats and displays the object’s XML text. // Format the XML text.StringWriter stringwriter = new StringWriter;XmlTextWriter xmltextwriter = new XmlTextWriter(stringwriter);xmltextwriter.Formatting = Formatting.Indented;xmldocument.WriteTo(xmltextwriter);// Display the result.txtResult.Text = stringwriter.ToString;This code creates a StringWriter and an XmlTextWriter associated with it. It sets the XmlTextWriter‘s properties so it formats the XML code with indentation and then calls the XML document object’s WriteTo method to write the document’s XML code into the XmlTextWriter (which writes it into the StringWriter). Finally, the code displays the text contained in the StringWriter.The following text shows the result.
Save Xml File From String C#
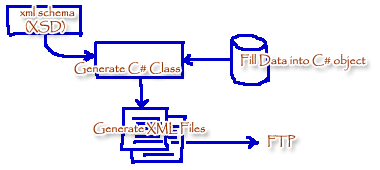
It's possible that I've missed things, but this all looks pretty good. The things that I'd highlight are that you have functions that can fail that are returning void. So for your void writeOpenTag(const std::string);void writeCloseTag;void writeStartElementTag(const std::string);void writeEndElementTag;void writeAttribute(const std::string);void writeString(const std::string);I'd make them either int or bool, depending on how you're feeling, and if you fail to open the file, or the file is already open, return a fail code, else return success, and have this in place of your cout. Coding style tidbits:The first thing that bothers me in your code is the use of unnamedfunction parameters in the header declaration. I consider that to be a bad practice becausethe name of the parameter itself is a form of code documentation.
C# Save Class As Xml
You shouldprovide the parameter names in the header file as well as in the source.Your comments in the.cpp are quite verbose. Seems like you are followingsome documentation template. Most experienced programmers will dislike it. Youshould of course comment and document the code, but do it in a meaningful manner,not just in an automated fashion. Things that are too obvious don't need to becommented.To make my point clear, take this line for example: // fileName const std::string The name of the file that is in useWell, it is implicit that fileName is 'The name of the file that is in use',so this should not be commented.If there are any caveats about the filename, however, then it must be commented.For example, does the filename have to have a.xml extension at the end? What happens if it doesn't?This is the sort of things you should be commenting.Missing constructors:There is a thing in C which is called the (or five/zero). It isabout how an object behaves regarding copy via constructors and assignment with operator =.Also, regarding constructors, I would expect to see a constructor that takesthe filename and opens the file for me without having to explicitly call open.This would be nice: XmlWriter xml('test.xml');// ready to go if successful, an exception otherwise.Error handling:There were some other suggestions about possible alternatives to do moreerror handling in your writer.
Error codes are somewhat OK, depending on thecontext. However, in a modern C library, as it seems to be the case with your code,exceptions are preferred for error reporting/handling.When writing files, there is only a limited set of errors thatcan happen. An IO/Write error is very unlikely on modern hardware, so an exceptionseems like a very nice fit for such cases.
How To Save Xml File In Project Folder Using C#
Use exceptions for exceptional things.So I'd vote against error codes and would suggest that you define a customexception type (derived from ) that your class throws on exceptionalcircumstances (file not open, IO error, etc). E.g.: an XmlWriterException type.String parameters passed by const value:When passing complex objects as function parameters, the worst possible wayto do it is by const value. Const disables move, so the compiler must issuea copy, even if you are just reading the param. If you then copy it to somestorage inside the function, you end up doing two copies.A good rule of thumb when passing objects as params is this:.If you are only reading the parameter, pass it by const reference ( const T &).If you are going to copy that object to somewhere inside the function,then pass it by value and apply on it.Improvements to exists:An fstream is not ideal for just checking if a file exists. But actually,XmlWriter::exists will ALWAYS succeed, because the default openflags of fstream are iosbase::in iosbase::out.So it will create a new file if it doesn't yet exists.You should be using if your purpose is to only test ifa file exists.cout for error reporting:This not a good choice for error logging/reporting. If you do implementexceptions, then the error message should be stored inside the exceptionobject. Otherwise, cout is for normal program output.
For error reporting,you use (STDERR).